Definition #
Dynamic variables are variables whose values can be changed at runtime, meaning their values can be updated during the execution of the program. In other words, the value of a dynamic variable is not fixed or predetermined at the time of declaration and you can use it to capture, modify and pass data between different sections of RTILA automation flows. Dynamic variables in JavaScript are particularly useful for storing and updating data that may change during the execution of a program, such as a user input or the results of a computation.
Objective #
The primary purpose of this command is to let you write and run your own custom JavaScript code directly inside the browser context. You can input your code into the Dynamic Variable command field, and RTILA will execute it in the target page. The result of your script will be automatically stored in a variable named after your command. You can then reuse this result in any subsequent RTILA command or flow by referencing it with the {{MyVariableName}}
syntax.
How to add a Dynamic Variable to your flow #
To add the Dynamic variable, you will need to go to the variables tab of a new command interface and search for it. After finding the variable and adding it, you can edit its value and set some other configurations.
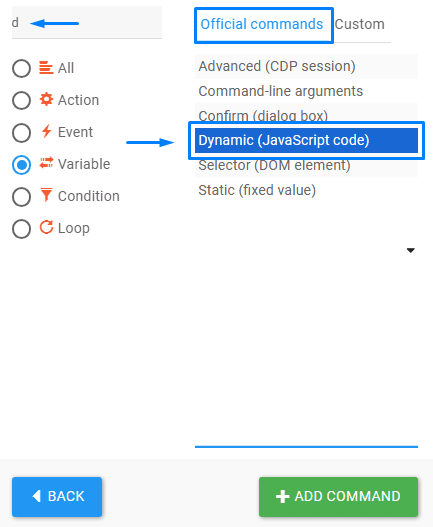
Configure a Dynamic Variable #
To add a dynamic variable, you will need to go to the variables tab of a new command interface and search for it. After finding the variable and adding it, you can edit its value and set some other configurations.
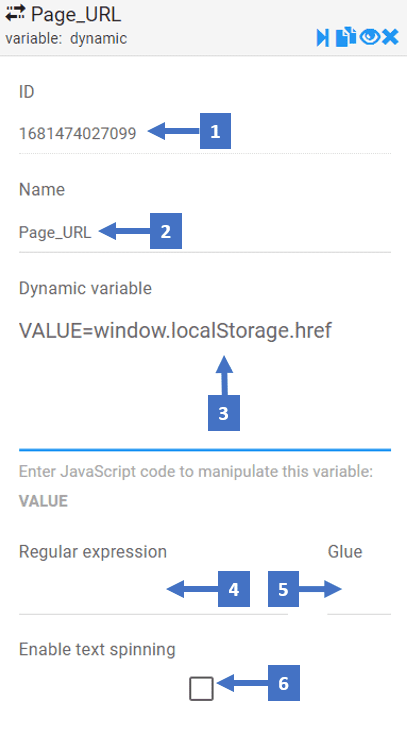
- This refers to the ID of the variable which you can call later to get the value of this variable
- Edit the name of the dynamic variable describing what value it would contain
- Here you can enter a javascript code that contains the returned value of the dynamic variable regaining with “VALUE=” for example VALUE=window.location.href
- A sequence of characters that forms a search pattern. For example, you can use regex to find all email addresses in a document or to extract all the numbers in a string.
- This allows you to combine different pieces of data or text together
- Enabling text spinning in a static var means allowing a static variable to store multiple versions of the same text, each with different wordings and phrasings, so that when the variable is called in a program or script, a different version of the text is returned each time
Dynamic Variable In Action #
Here is an example of using “Dynamic Variable ” with JavaScript code to get the current URL of a page and call the variable data (in this case the current page URL) in an alert message
Useful JavaScript code lines for Dynamic Variable #
Applicability to Properties values #
The shared JavaScript lines of codes can also be used for Properties as a Filter > Actions. The only difference is that you need replace every instance of VALUE by FIELD_VALUE when used on the Property side. For instance instead of VALUE=VALUE.replace(‘allXX’,’byYY’ ); you would use FIELD_VALUE=FIELD_VALUE.replace(‘allXX’,’byYY’ );
🧪 Debug Tip: Log VALUE before returning
At the bottom of your custom JS code just add this line:
console.log(“VALUE:”, VALUE);
This helps you check if your script is producing the expected value inside the browser.
console.log("VALUE:", VALUE);
Common Examples of Dynamic JS Code #
Get Current URL #
VALUE=window.location.href
console.log("VALUE:", VALUE);
Replace strings in current variable value #
VALUE=VALUE.replace('allXX','byYY' );
console.log("VALUE:", VALUE);
Prepend current variable value #
VALUE = "YourTextHere" + VALUE;
console.log("VALUE:", VALUE);
Append current variable value #
VALUE = `${VALUE}YourTextHere`;
console.log("VALUE:", VALUE);
Concatenate other variable commands values #
VALUE = '{{DOMSelectorVariable}}'+'{{StaticVariable}}'+'{{DynamicVariableX}}';
console.log("VALUE:", VALUE);
Sanitize other variable command values #
VALUE = '{{DOMSelectorVariable}}'.toLowerCase().replace(/[^a-z]/g, '');
console.log("VALUE:", VALUE);
Get The Current Date #
let currentDate = new Date();
let year = currentDate.getFullYear();
let month = currentDate.getMonth() + 1; // add 1 to get the actual month (0-based index)
let day = currentDate.getDate();
VALUE = `Today's date is ${year}-${month}-${day}`
console.log("VALUE:", VALUE);
Get The Current Date & Time #
let currentDate = new Date();
let year = currentDate.getFullYear();
let month = ('0' + (currentDate.getMonth() + 1)).slice(-2);
let day = ('0' + currentDate.getDate()).slice(-2);
let hours = ('0' + currentDate.getHours()).slice(-2);
let minutes = ('0' + currentDate.getMinutes()).slice(-2);
let seconds = ('0' + currentDate.getSeconds()).slice(-2);
VALUE= 'Date / '+ year + '-' + month + '-' + day + ' Time /' + hours + ':' + minutes + ':' + seconds;
console.log("VALUE:", VALUE);
Get The Current Value of a LocalStorage Variable #
VALUE=window.localStorage.getItem('Replace this with your local storage variable');
console.log("VALUE:", VALUE);
Get TimeStamp In Seconds #
VALUE = Date.now();
console.log("VALUE:", VALUE);
Get The Current IP Address #
VALUE= fetch('https://api.ipify.org?format=json').then(response => response.json()).then(data => FIELD_VALUE = data.ip).catch(error => console.error('Error fetching public IP address:', error));
console.log("VALUE:", VALUE);
RTILA Dynamic JS Code Command (Advanced Guide) #
The Dynamic JS Code command in RTILA allows you to execute custom JavaScript code inside a page and return a result into a dynamic variable (e.g., {{MyVariable}}
). This is a powerful feature for scraping, automation, conditional logic, and data extraction — even from complex or Shadow DOM elements.
📌 What This Command Does #
- Executes JavaScript code inside the target page context (browser-side).
- Supports asynchronous operations like delays, button clicks, and tab handling.
- Stores the final result into a variable that can be reused in the next steps using
{{MyVariable}}
.
🛠️ How It Works Internally #
Under the hood, the command runs your JS code like this:
commandValues = await page.evaluate(async function (jsCode) {
let VALUE;
const asyncCode = `(async () => {
${jsCode}
return typeof VALUE !== 'undefined' ? VALUE : 'VALUE_NOT_SET';
})()`;
return await eval(asyncCode);
}, command.dynamicVariable);
💡 Important: #
VALUE
is a reserved keyword in RTILA — it is used to pass your result into the dynamic variable.
Do NOT declare VALUE using let, const, or var. The VALUE
variable is already pre-declared by RTILA in the evaluation scope.
Your custom JavaScript code should only assign a value to it, like this:
VALUE = 'final result';
✅ Basic Example (Extract Page Title) where result {{MyVariable}}
will contain and output the saved page title.
VALUE = document.title;
✅ Advanced Example: Click in Shadow DOM & Extract New Tab URL #
const delay = ms => new Promise(res => setTimeout(res, ms));
const host = document.querySelector('li:nth-of-type(1) svelte-component[type="ProfileCard"]');
if (!host) throw new Error("ProfileCard host not found.");
const shadowRoot = host.shadowRoot;
if (!shadowRoot) throw new Error("Shadow root not accessible.");
const linkedinButton = shadowRoot.querySelector('button[data-testid="social-button"] i.fa-linkedin-in');
if (!linkedinButton) throw new Error("LinkedIn button not found.");
const button = linkedinButton.closest('button');
if (!button) throw new Error("Button wrapper not found.");
let newTab = null;
const originalOpen = window.open;
window.open = function (...args) {
newTab = originalOpen.apply(this, args);
return newTab;
};
button.click();
await delay(5000);
window.open = originalOpen;
let finalUrl = 'cross-origin-or-unknown';
try {
if (newTab && newTab.location && newTab.location.href) {
finalUrl = newTab.location.href;
}
} catch (e) {
finalUrl = 'cross-origin-or-unknown';
}
newTab.close();
VALUE = finalUrl;
console.log("VALUE:", VALUE);
🧩 Best Practices
Do | Don’t |
---|---|
✅ Always assign your final result to VALUE | ❌ Don’t wrap VALUE assignment inside try-catch unless handled properly |
✅ Use await for async actions like delays | ❌ Don’t assume window.open() will always be same-origin |
✅ Console.log VALUE for debugging | ❌ Don’t re-declare VALUE inside a different scope |
✅ Test from DevTools Console before inserting | ❌ Avoid eval() inside your code — it’s already used by RTILA |
⚠️ Common Pitfalls #
🔁 Problem: {{MyVariable}}
is blank or crashes RTILA #
Cause: Your code didn’t assign VALUE
in the correct (top-level) scope.
Fix: Ensure this line runs in the main body, not inside another function or suppressed try-catch
:
VALUE = yourResult;
🧪 Reminder Tip: Log VALUE before returning
This helps you check if your script is producing the expected value inside the browser.
console.log("VALUE:", VALUE);
🧰 Use Cases #
- Extract data from dynamic or Shadow DOM
- Wait for page elements before acting
- Interact with modals, dropdowns, and iFrames
- Capture data from newly opened tabs
- Inject conditional logic before proceeding