This guide explores how to manage arrays and objects within your RTILA automation. You’ll learn how to leverage Dynamic Variable (JavaScript code) command to manipulate data structures similar to how you would in JavaScript.
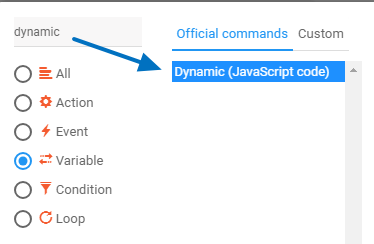
We’ll use the JSONPlaceholder API to retrieve sample data. This API provides a convenient way to access demo user information. You can explore the type of data available by clicking this link/endpoint: https://jsonplaceholder.typicode.com/users.
Here’s a sample JavaScript code snippet that fetches user data asynchronously:
async function getUsers() {
try {
const response = await fetch('https://jsonplaceholder.typicode.com/users');
if (!response.ok) {
throw new Error(`Error fetching users: ${response.status}`);
}
const data = await response.json();
return JSON.stringify(data);
console.log(data);
} catch (error) {
console.error('Error:', error);
}
}
VALUE=getUsers();
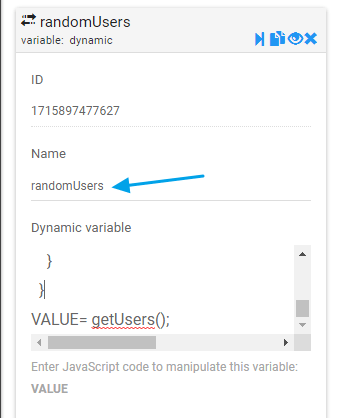
So we can now access and manipulate that array anywhere throughout your automation, let’s say you need to save the name of the first user that you would later need to use in the flow or extract in the results. with using the dynamic variable, you can achieve it like thisVALUE={{randomUsers}}[0].name
; // Access the first object ([0]) in the array and then its "name" property
so here we are basically accessing the first object in the array and then the name of the user.
Now we can extract that name with using the variable name {{firstuser_name}}
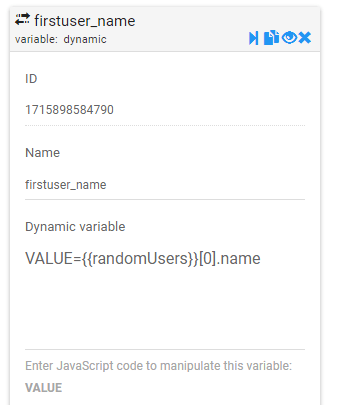
This approach works for accessing any other piece of information within the fetched data. The structure you used to extract the name can be applied to retrieve other properties of the user objects or elements from the array.
here is the link to the rtila project template
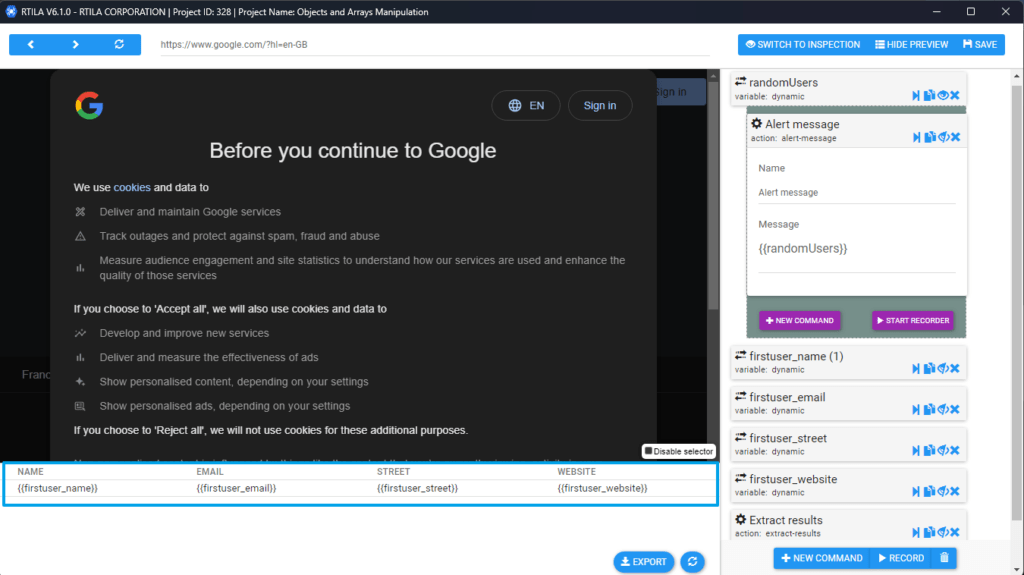